This is a living document. I plan to update it often with new problems and solutions.
Preparing for technical interviews time consuming and difficult. To hold myself accountable, I am uploading all my solutions along with explanations in this article. I hope you enjoy!
2114. Maximum Number of Words Found in Sentences
Question on Leetcode.
Question
A sentence is a list of words that are separated by a single space with no leading or trailing spaces. You are given an array of strings sentences, where each sentences[i] represents a single sentence. Return the maximum number of words that appear in a single sentence.
Solutions
JavaScript
var mostWordsFound = function(sentences) {
let maxCount = sentences[0].split(" ").length
// loop over each sentence
for (let i = 1; i < sentences.length; i++) {
let tempCount = sentences[i].split(" ").length
if (tempCount > maxCount)
maxCount = tempCount
}
return maxCount
};
C#
public class Solution {
public int MostWordsFound(string[] sentences) {
int maxCount = sentences[0].Split(" ").Length;
foreach(string sentence in sentences.Skip(1)) {
int tempCount = sentence.Split(" ").Length;
if (tempCount > maxCount)
maxCount = tempCount;
}
return maxCount;
}
}
Java
class Solution {
public int mostWordsFound(String[] sentences) {
int total = 0;
for(int i = 0; sentences.length > i; i++){
int tempLength = sentences[i].split(" ").length;
if(tempLength > total){
total = tempLength;
}
}
return total;
}
}
Explanation
We are asked to figure out which sentence has the most words. The sentences are held in an array. The idea is to iterate over each of the sentences and count the number of words. Each word is seperated by a space. An easy way to get this count is to use split()
. After we split by spaces, we can use length
to get the count.
Each time we get the count, we should compare it to our previous count. Take the higher count. Initially, we will set the maxCount
to the first sentence. We can get this value like such:
let maxCount = sentences[0].split(" ").length
Then, we start our for loop at i = 1
.
Runtime
The runtime for this problem would be O(n)
where n is the number of sentences.
Submission Image
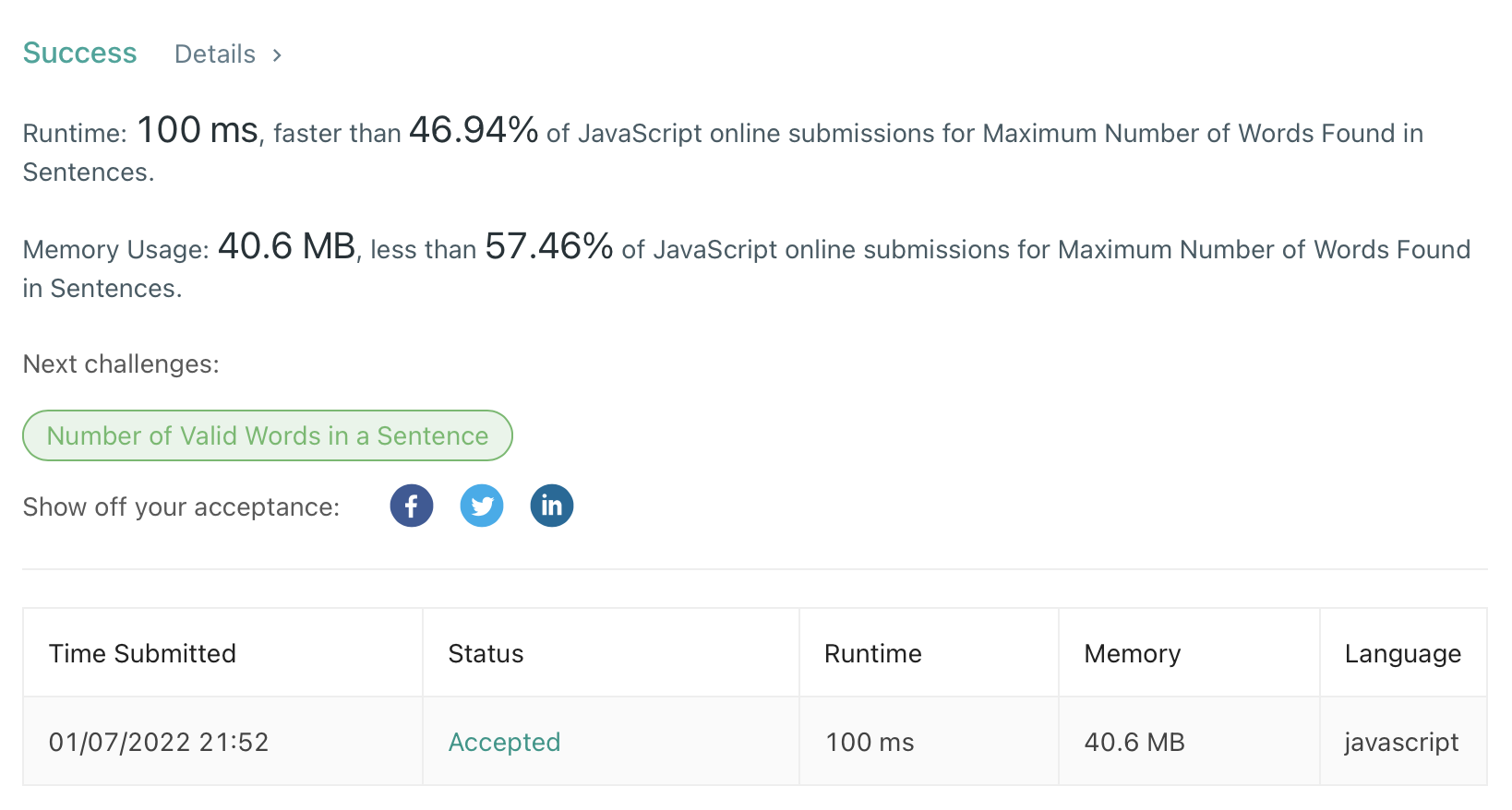
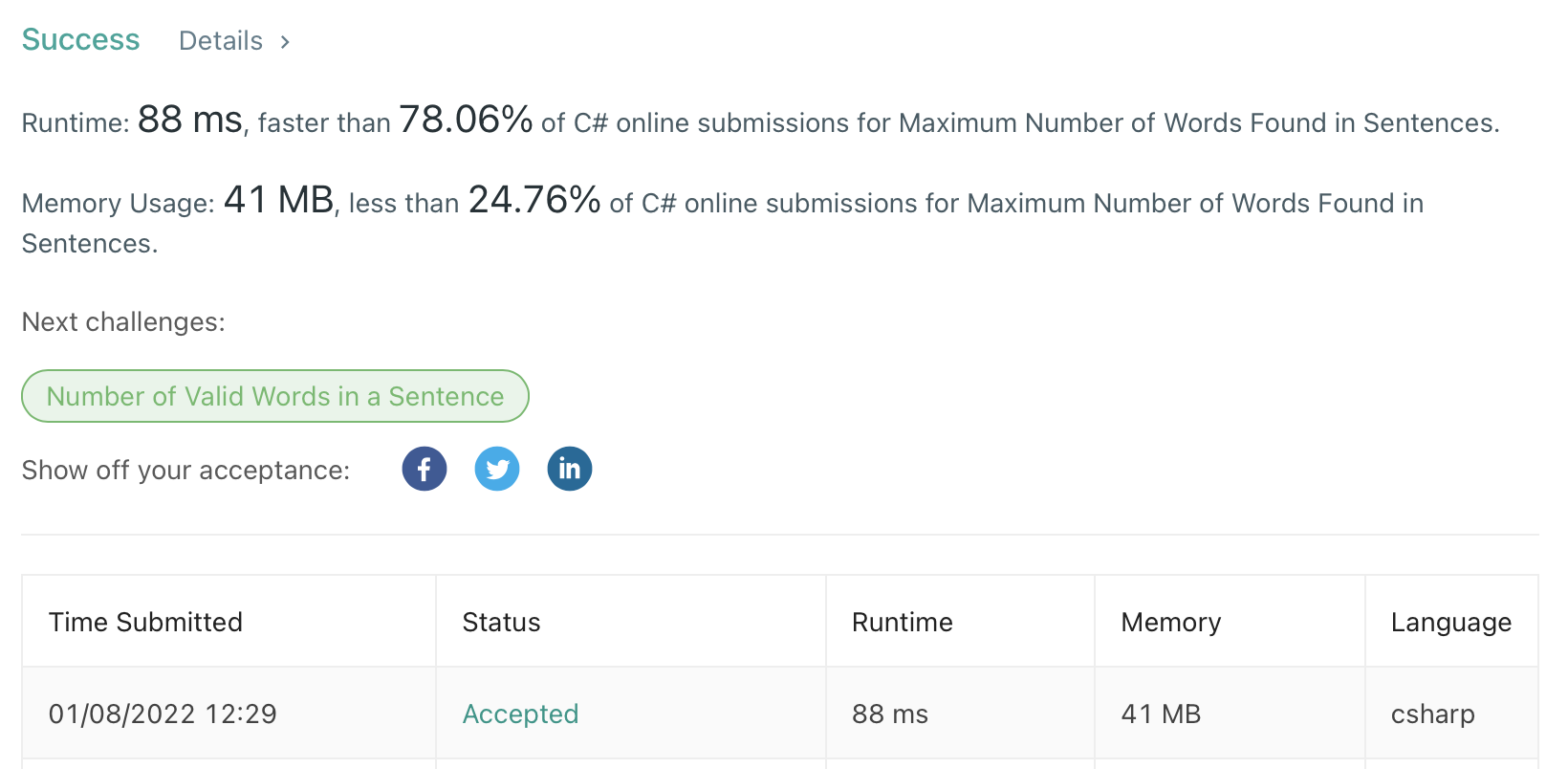
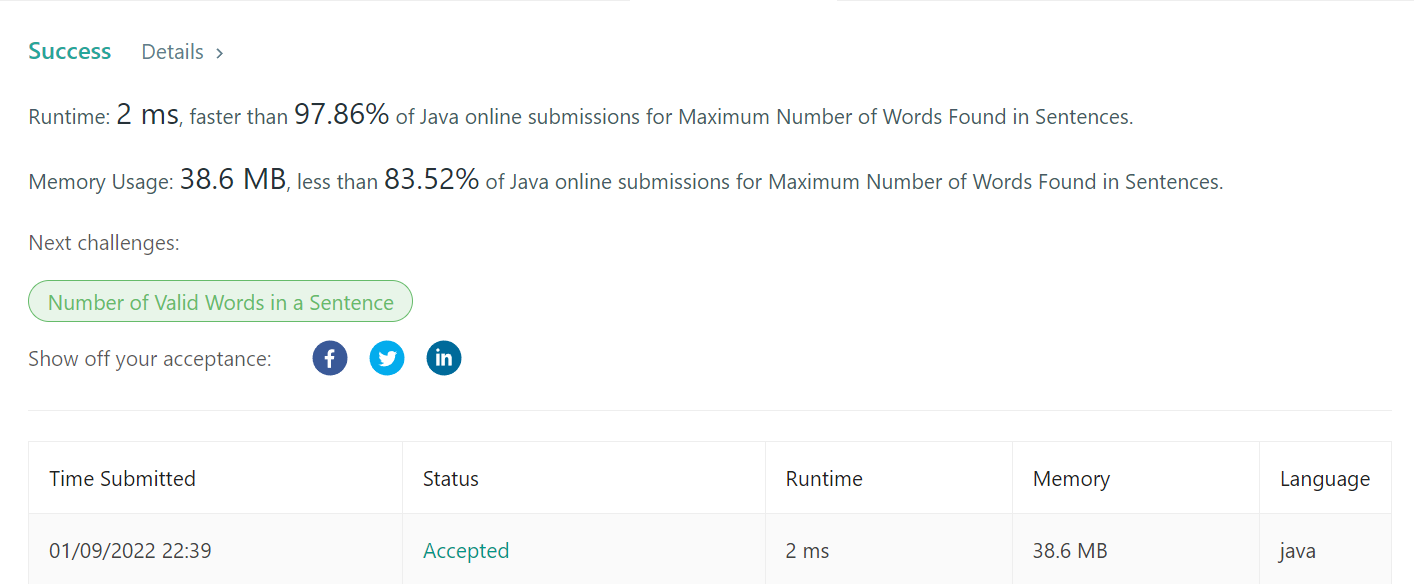